Application's Introduction of JDK8's Lambda Syntax with Map
Author/MinCong Wu [Issue Date: 2015/1/5]
Oracle's JDK 8 added many new features and improved performance. This paper describes some simple methods of Lambda syntax with Map, making program code more streamlined and easy to read.
1. forEach
Let's take stock for example. Currently, each stock has one corresponding Map used to store the transaction price in the market. Before JDK8, to show the transaction price of stock, we must use the following two methods:
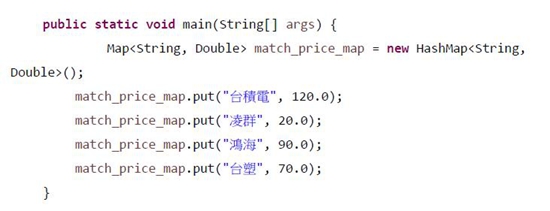
(1)
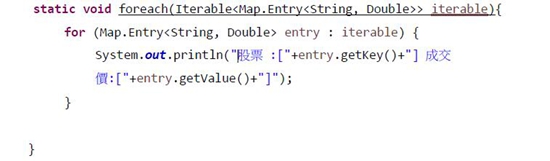
This method mainly uses the entry set to return the entry set of Map and then uses the "for loop" to search the set for showing each key and value. In this method, the readability of the program is very poor.
(2)
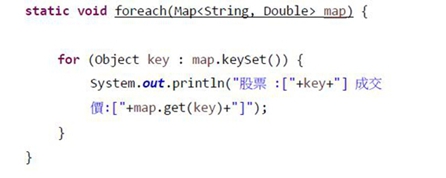
This method mainly uses map.keySet () to get each key, and then uses this Key to get the corresponding Value in Map. Compared with method (1), the readability of this program is much better.
(3)JDK8:

This method combines JDK8 and Lamdba expression. You can simply search Keys and Values which are in Map, while also taking into account the readability of the program.
Execution results are as follows:
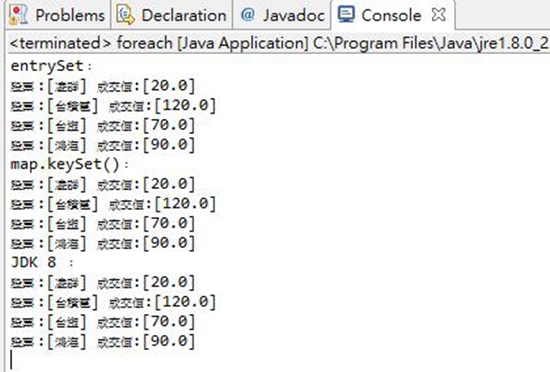
2. getOrDefault and putIfAbsent
Formerly, if a value does not exist, the default value must be set while getting a Key value. When operating the Map, if there is no corresponding Key value in Map, it will return Null. In this case, if accessing the return value directly, the null point exception will occur. In order to avoid this situation, we usually use the following methods to determine whether the obtained contents are empty.
From the above examples, we can see that only when the stock has been sold the transaction price will be stored in Map. When Map cannot get the transaction price it will be displayed today has no turnover, and will use -1 to express the situation.
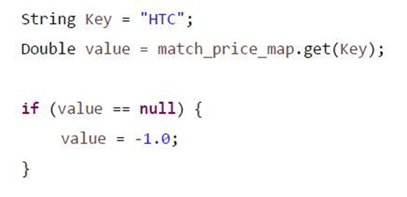
But in JDK8, a new Method was added to do this.

If you cannot get Values, but you want to save the default value to Map, you can use the following command.

Finally, showing the transaction price of all stock:
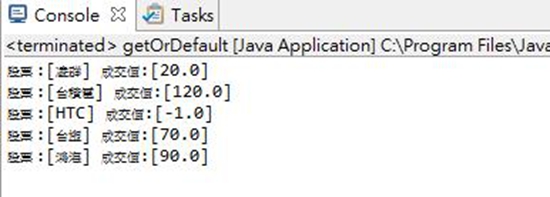
3. Compute
This method provides the specified Key and Lambda operations to determine the Value. Continuing with the previous example, now when calculating the closing stock price, if the day's closing price is based on the day's last transaction price and there's no turnover, the previous day's closing price is used for the calculating.
Suppose the previous day's closing price is as follows:
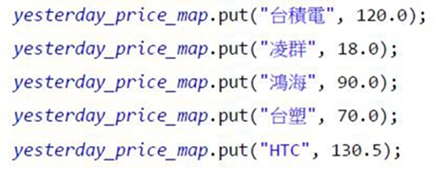
The calculation method of closing price is as follows:
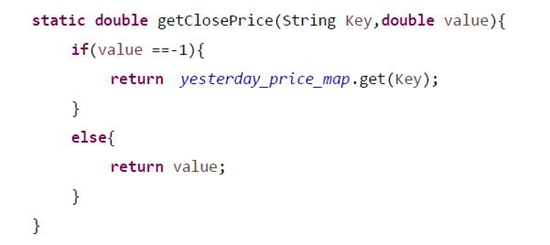
Then you can use the following method to calculate the closing price of all stocks by computing:
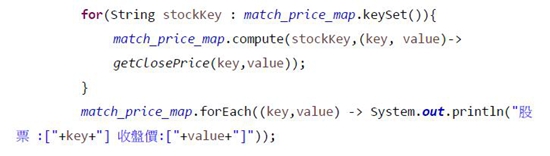
Execution results are as follows:
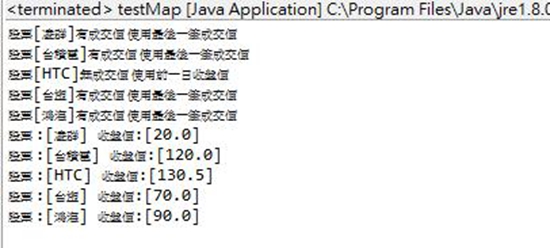
According to the results, we find "match_price_map.compute (stockKey, (key, value) -> getClosePrice (key, value));". The operation takes out each Key and Value in Map which stored the transaction price, and storing the closing price to Map by getClosePrice operation and using the Key:[HTC] to explain that since there was no transaction price we'll use the previous day's closing price from yesterday_price_map and put it into the original Map as today's closing price.
4. computeIfAbsent
Now we use the Fibonacci series to illustrate the convenience of computeIfAbsent method. The definition of the Fibonacci series is as follows.
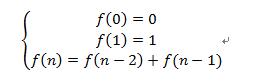
When not using map, you can calculate the value which corresponds to the answer by recursion. The program code is as follows.
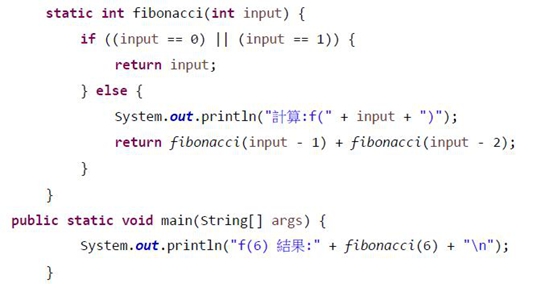
Execution results are as follows:
Calculation:f(6)
Calculation:f(5)
Calculation:f(4)
Calculation:f(3)
Calculation:f(2)
Calculation:f(2)
Calculation:f(3)
Calculation:f(2)
Calculation:f(4)
Calculation:f(3)
Calculation:f(2)
Calculation:f(2)
The result of f(6) is 8.
According to the results of the calculation, we see that when not using Map, to get the results of f(6), we must calculate 12 times and many values will be double counted. But when using Map, the number of double-counts is reduced, as follows:
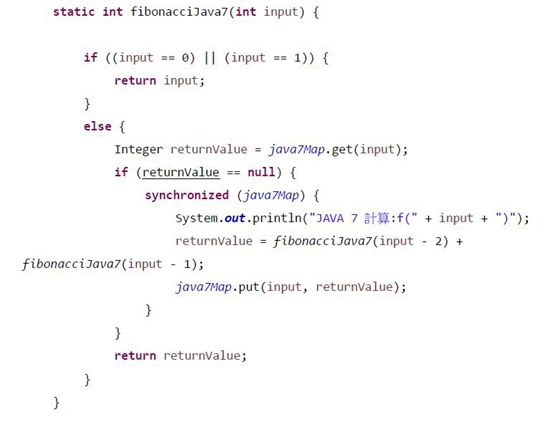
Execution results are as follows:
JAVA 7 Calculation: f(6)
JAVA 7 Calculation: f(4)
JAVA 7 Calculation: f(2)
JAVA 7 Calculation: f(3)
JAVA 7 Calculation: f(5)
The result of JAVA 7 f(6) is 8.
According to the results of the calculation, we see that the number of calculations is reduced from 12 to 5 and double-counting has been eliminated.
Using JDK8 we can shorten the program code using computeIfAbsent with Lambda expressions. ComputeIfAbsent performs the specified operation when the Key in Map does not have a corresponding Value. The result is put into Map and returned.
The program code is as follows:
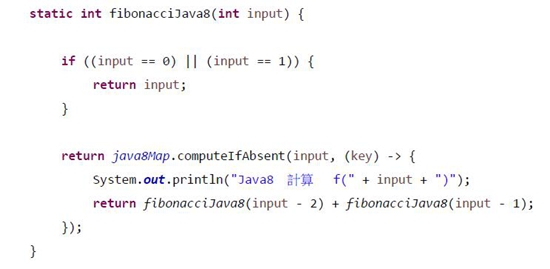
Execution results are as follows:
Java8 Calculation: f(6)
Java8 Calculation: f(4)
Java8 Calculation: f(2)
Java8 Calculation: f(3)
Java8 Calculation: f(5)
The result of JAVA8 f(6) is 8.
Based on the example and execution results, there is less program code when using the computeIfAbsent as compared to JDK 7 and consider the performance advantage also.
Conclusion
JDK 8 most significant change is the introduction of the Lambda expression and newly added APIs. These reduce the necessary program code and improve code readability. JDK 8 also has many new features, such as a new JavaScript engine [Nashorn], new Stream API, security enhancements, and more. All of these provide convenient methods for programmers to design programs. There are many more new functions that we leave for the reader to explore themselves.